A Comprehensive List of Python in Excel Resources
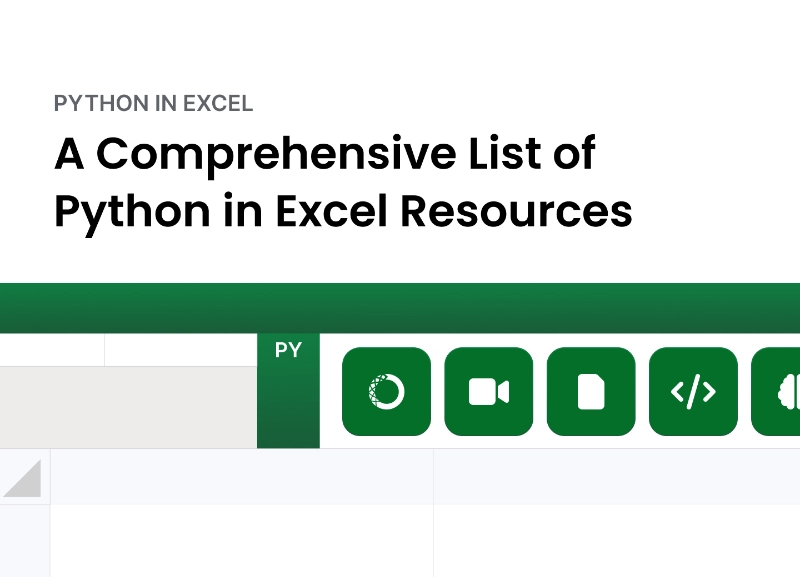
Andrew Huang and Sophia Yang
Andrew Huang and Sophia Yang
With its latest version 1.3, the open-source project Panel has just introduced an exciting and highly anticipated new feature: the Chat Interface widget. This new capability has opened up a world of possibilities, making the creation of AI chatbots more accessible and user-friendly than ever before.
In this post, you’ll learn how to use the ChatInterface widget and to build:
Before we get started, you will need to install Panel (any version greater than 1.3.0) and other packages you might need like jupyterlab, openai, and langchain.
Now you are ready to go!
The brand new ChatInterface widget is a high-level widget, providing a user-friendly chat interface to send messages with four build-in operations:
Curious to know more about how `ChatInterface` works under the hood? It’s a high-level widget that wraps around the middle-level widget `ChatFeed` that manages a list of `ChatMessage` items for displaying chat messages. Check out the docs on ChatInterface, ChatFeed and ChatMessage to learn more.
With `pn.chat.ChatInterface`, we can send messages to the chat interface, but how should the system reply? We can define a `callback` function!
In this example, our `callback` function simply echoes back a user message. See how it’s becoming more functional already?
How to use the ChatInterface widget to echo back a message:
import panel as pn
pn.extension()
def callback(contents: str, user: str, instance: pn.chat.ChatInterface):
message = f"Echoing {user}: {contents}"
return message
chat_interface = pn.chat.ChatInterface(callback=callback, callback_user="System")
chat_interface.send("Send a message to receive an echo!", user="System", respond=False)
chat_interface.servable()
To serve the app, run panel serve app.py
or panel serve app.ipynb
.
How can we use OpenAI ChatGPT to reply to messages? We can simply call the OpenAI API in the `callback` function.
Install `openai` in your environment and add your OpenAI API key to the script. Note that in this example, we added `async` to the function to allow collaborative multitasking within a single thread and allow IO tasks to happen in the background. This ensures that our app runs smoothly while waiting for OpenAI API responses. Async enables concurrent execution, allowing us to perform other tasks while waiting and ensuring a responsive application.
How to use the ChatInterface widget to create a chatbot using OpenAI’s GPT-3 API:
import openai
import panel as pn
pn.extension()
openai.api_key = "Add your key here"
async def callback(contents: str, user: str, instance: pn.chat.ChatInterface):
response = openai.ChatCompletion.create(
model="gpt-3.5-turbo",
messages=[{"role": "user", "content": contents}],
stream=True,
)
message = ""
for chunk in response:
message += chunk["choices"][0]["delta"].get("content", "")
yield message
chat_interface = pn.chat.ChatInterface(callback=callback, callback_user="ChatGPT")
chat_interface.send(
"Send a message to get a reply from ChatGPT!", user="System", respond=False
)
chat_interface.servable()
The Panel ChatInterface also seamlessly integrates with LangChain, leveraging the full spectrum of LangChain’s capabilities.
Here is an example of how we use LangChain’s `ConversationChain` with the `ConversationBufferMemory` to store messages and pass previous messages to the OpenAI API.
Again, please remember to make sure to install `langchain` in your environment and add your OpenAI API key in the script.
Note that before we dive into the LangChain code, we defined a `callback_handler` because LangChain interface does not have a way to stream from generators, so we need to wrap the LangChain interface with `pn.chat.langchain.PanelCallbackHandler`, which inherits from `langchain.callbacks.base.BaseCallbackHandler`. For more information, check out the documentation.
How to use the ChatInterface widget to create a chatbot using OpenAI’s GPT-3 API with LangChain:
import os
import panel as pn
from langchain.chat_models import ChatOpenAI
from langchain.chains import ConversationChain
from langchain.memory import ConversationBufferMemory
pn.extension()
os.environ["OPENAI_API_KEY"] = "Type your API key here"
async def callback(contents: str, user: str, instance: pn.chat.ChatInterface):
await chain.apredict(input=contents)
chat_interface = pn.chat.ChatInterface(callback=callback, callback_user="ChatGPT")
callback_handler = pn.chat.langchain.PanelCallbackHandler(chat_interface)
llm = ChatOpenAI(streaming=True, callbacks=[callback_handler])
memory = ConversationBufferMemory()
chain = ConversationChain(llm=llm, memory=memory)
chat_interface.send(
"Send a message to get a reply from ChatGPT!", user="System", respond=False)
chat_interface.servable()
In this blog post, we’ve taken an in-depth look at the exciting new ChatInterface widget in Panel. We started by guiding you through building a basic chatbot using `pn.chat.ChatInterface`. We elevated your chatbot’s capabilities from there by seamlessly integrating OpenAI ChatGPT. To further enhance your understanding, we also explored the integration of LangChain with Panel’s ChatInterface. If you’re eager to explore more chatbot examples, don’t hesitate to visit this GitHub repository and consider contributing your own.
Armed with these insights and hands-on examples, you’re now well-prepared to embark on your journey of crafting AI chatbots in Panel. Happy coding!
All of these tools are open source and free for everyone to use, but if you’d like to get started with help from Anaconda’s AI and Python app experts, contact a representative from our sales team at [email protected].
Talk to one of our experts to find solutions for your AI journey.